Introduction to Oracle Web Service Manager
Oracle Web Services Manager (OWSM) is a Web services security and management solution that provides a common security infrastructure for all Web service applications. It provides an out-of-the-box (readily available with the product) common security policies (such as authentication, authorization, message encryption, message signing, and so on). This helps us to implement security for web services quickly without interfering with service implementation.
OWSM is a component of SOA Suite. It provides centralized policy management for governing SOA interactions. It provides visibility and control of the policies through a centralized administration interface offered by Oracle Enterprise Manager.
Key Benefits
- Establish trust – Build security and operation level policies that can be layered over new or existing applications and services.
- Automate consumer management – Automate enforcement of contracts between consumer and provider to preserve business alignment.
- Ensure service levels – Use dashboards to monitor policies as they execute, to ensure service levels and avoid potential problems.
- Minimize compliance risk – Centralize creation and management of policies and apply anywhere.
- Policies can be attached and detached at runtime by the administrators.
What is OWSM Repository
- Oracle Web Services Manager (WSM) uses an MDS repository to store OWSM metadata, such as policies, assertion templates, and policy usage data.
- OWSM Repository is available as a database (for production use) or as files in the file system (for development use in JDeveloper).
Custom OWSM Policy & Example Implementation
We can implement custom policies when out of the box policies don’t meet our needs. This blog describes how to develop a simple custom policy to demonstrate the capability. Depending on the type and configuration of the policy, they can be triggered at different levels and perform different tasks. These policies do not interfere with the internals of a core service. A Policy can be composed of a number of “Assertions”. Typically, you will have at least one Assertion in a Policy.
This example, IP Address Validation Custom Assertion Policy, validates whether a request that is made to the Web service is from a set of valid IP addresses.
To make it simple, I am keeping the list of IP address as comma separated value in the policy. Any request coming from other IP address results in a FAILED_CHECK response.
The steps described in this blog to create and put the custom policy into action are:
- Create Custom Policy:
Custom Assertion Executor Class
Policy Executor XML File
Policy Configuration XML File
- Deploy & Import Policy Artifacts to Fusion Middleware Runtime platform
- Attach Policies to services
- Check the results produced by the policy attachment
Custom Assertion Executor Class
Create java class which Implements oracle.wsm.policyengine.impl.AssertionExecutor..
Implement the custom assertion executor method to validate the IP addresses (logic of your policy assertion).
When building the custom assertion executor, you will need wsm-policy-core.jar, wsm-agent-core.jar in your classpath which are located at oracle_common/modules/oracle.wsm.common_12.1.3 for 12.1.3 version and for 12.2.1 at oracle_common/modules/oracle.wsm.common.
Please refer the Oracle blog for more information.
Example Custom Assertion Executor:
package fd.custom.owsmcustompolicy; import oracle.wsm.common.sdk.IContext; import oracle.wsm.common.sdk.IMessageContext; import oracle.wsm.common.sdk.IResult; import oracle.wsm.common.sdk.Result; import oracle.wsm.common.sdk.WSMException; import oracle.wsm.policy.model.IAssertion; import oracle.wsm.policy.model.IAssertionBindings; import oracle.wsm.policy.model.IConfig; import oracle.wsm.policy.model.IPropertySet; import oracle.wsm.policy.model.ISimpleOracleAssertion; import oracle.wsm.policy.model.impl.SimpleAssertion; import oracle.wsm.policyengine.IExecutionContext; import oracle.wsm.policyengine.impl.AssertionExecutor; public class CustomPolicyAssertion extends AssertionExecutor { public CustomPolicyAssertion() { super(); } @Override public IResult execute(IContext Context) throws WSMException { try { //Retrieve Plociy bindings from Policy File IAssertionBindings bindings = ((SimpleAssertion) (this.assertion)).getBindings(); //Get Plociy Config name from Policy File IConfig config = bindings.getConfigs().get(0); //Get Property set name of policy IPropertySet propertyset = config.getPropertySets().get(0); String valid_ips = propertyset.getPropertyByName("valid_ips").getValue(); String ipAddr = ((IMessageContext) Context).getRemoteAddr(); IResult result = new Result(); if (valid_ips != null && valid_ips.trim().length() > 0) { String[] valid_ips_array = valid_ips.split(","); boolean isPresent = false; for (String valid_ip: valid_ips_array) { if (ipAddr.equals(valid_ip.trim())) { isPresent = true; } } if (isPresent) { result.setStatus(IResult.SUCCEEDED); } else { result.setStatus(IResult.FAILED); result.setFault(new WSMException(WSMException.FAULT_FAILED_CHECK)); } } else { result.setStatus(IResult.SUCCEEDED); } return result; } catch (Exception e) { throw new WSMException(WSMException.FAULT_FAILED_CHECK, e); } } //The init() method is invoked by the OWSM framework whenever the configuration of the policy attachment is updated (i.e. its property values are changed). @Override public void init(IAssertion assertion, IExecutionContext econtext, IContext Context) throws WSMException { this.assertion = assertion; this.econtext = econtext; } public oracle.wsm.policyengine.IExecutionContext getExecutionContext() { return this.econtext; } public boolean isAssertionEnabled() { //return policy enforce value return ((ISimpleOracleAssertion) this.assertion).isEnforced(); } public String getAssertionName() { return this.assertion.getQName().toString(); } @Override public void destroy() { } public oracle.wsm.common.sdk.IResult postExecute(oracle.wsm.common.sdk.IContext p1) { IResult result = new Result(); result.setStatus(IResult.SUCCEEDED); return result; } }
Policy Executor File
<?xml version = '1.0' encoding = 'UTF-8'?> <wsp:Policy xmlns="http://schemas.xmlsoap.org/ws/2004/09/policy" xmlns:orasp="http://schemas.oracle.com/ws/2006/01/securitypolicy" orawsp:status="enabled" xmlns:wsu="http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" orawsp:category="security" orawsp:attachTo="binding.server" wsu:Id="ip_assertion_policy" xmlns:orawsp="http://schemas.oracle.com/ws/2006/01/policy" xmlns:wsp="http://schemas.xmlsoap.org/ws/2004/09/policy" wsp:Name="oracle/ip_assertion_policy"> <orasp:ipAssertion orawsp:Silent="true" orawsp:Enforced="true" orawsp:name="WSSecurity IpAssertion Validator" orawsp:category="security/authentication"> <orawsp:bindings> <orawsp:Implementation>fd.custom.owsmcustompolicy.CustomPolicyAssertion</orawsp:Implementation> <orawsp:Config orawsp:name="ipassertion" orawsp:configType="declarative"> <orawsp:PropertySet orawsp:name="valid_ips"> <orawsp:Property orawsp:name="valid_ips" orawsp:type="string" orawsp:contentType="constant"> <orawsp:Value>10.48.0.161</orawsp:Value> </orawsp:Property> </orawsp:PropertySet> </orawsp:Config> </orawsp:bindings> </orasp:ipAssertion> </wsp:Policy>
Policy Configuration XML File (Policy-Config.xml)
Create a policy-config.xml file that defines an entry for the new assertion and associates it with its custom assertion executor. You also need to specify the custom assertion executor class in the configuration file.
<?xml version="1.0" encoding="UTF-8"?> <policy-config> <policy-model-config> <entry> <key namespace="http://schemas.oracle.com/ws/2006/01/securitypolicy" element-name="ipAssertion"/> <executor-classname>fd.custom.owsmcustompolicy.CustomPolicyAssertion</executor-classname> </entry> </policy-model-config> </policy-config>
How to Build and Deploy (Import) OWSM Custom Policy Manually
Create a deployment profile (simple Java Archive) for the JDeveloper project. Deploy the project to a JAR file using this profile.
Copy JAR file to the WLS DOMAIN\lib directory and Restart the WebLogic domain.
Now Import Policy Definition into EM FMW Infrastructure and navigate to WSM Policies.
Click on Import to import a zip file with appropriate structure (this means it should contain a folder structure of META-INF\policies\some-custom-folder-name\policyname.xml.
The report back:
And the policy is listed:
Automate Build & Deploy (Import) Policy using FlexDeploy
To Automate through Flexdeploy follow the below steps to Build & Deploy Custom Policies.
- Build OWSM Custom Policy using Flexdeploy JDeveloper plugin operation “projectBuild”.
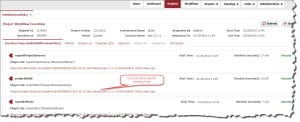
- Deploy custom policy using FlexDeploy having two options
- Deploy and Import OWSM Policy using FlexDeploy weblogic plugin.
- Import OWSM Policy using FlexDeploy weblogic plugin.
Deploy & Import OWSM Policy
This operation will copy the deployable jar file into WebLogic Domain Lib Directory automatically , Recycle the Admin Server and Import OWSM Custom Policies into a OWSM Repository. All in one step.
The below screenshot shows the FlexDeploy execution of DeployAndImportOWSMPolicy.
Evidence of Success
Below is the OWSM custom policy successfully imported into Web Logic domain OWSM Repository.
Import OWSM Policy
Imports OWSM Custom Policies into an OWSM Repository.
This operation assumes that the deployable jar file is already copied to the WebLogic Domain Lib Directory and the Admin server was already recycled. It only Imports the custom policy into OWSM Repository.
The below screenshot shows the FlexDeploy execution of Import OWSM Policy.
Evidence of Success
Below is the OWSM custom policy successfully imported into WebLogic domain OWSM Repository.